API Linkage
By using Chat&Messenger API, you can easily link with external systems and programs.
The API linkage enables the following processing.
- Linkage with internal systems and notification of business data to chat rooms
- Detects system errors and notifies relevant personnel
- Web conference linkage from internal groupware
- Obtain schedule and meeting room reservation information and link to other systems
API Token and API Interface
Obtaining an API Token from the administration screen
When performing API linkage, first obtain an API Token.
Pass the obtained apiToken / serverURL to JavaScript / curl as described in "API Interface" below.
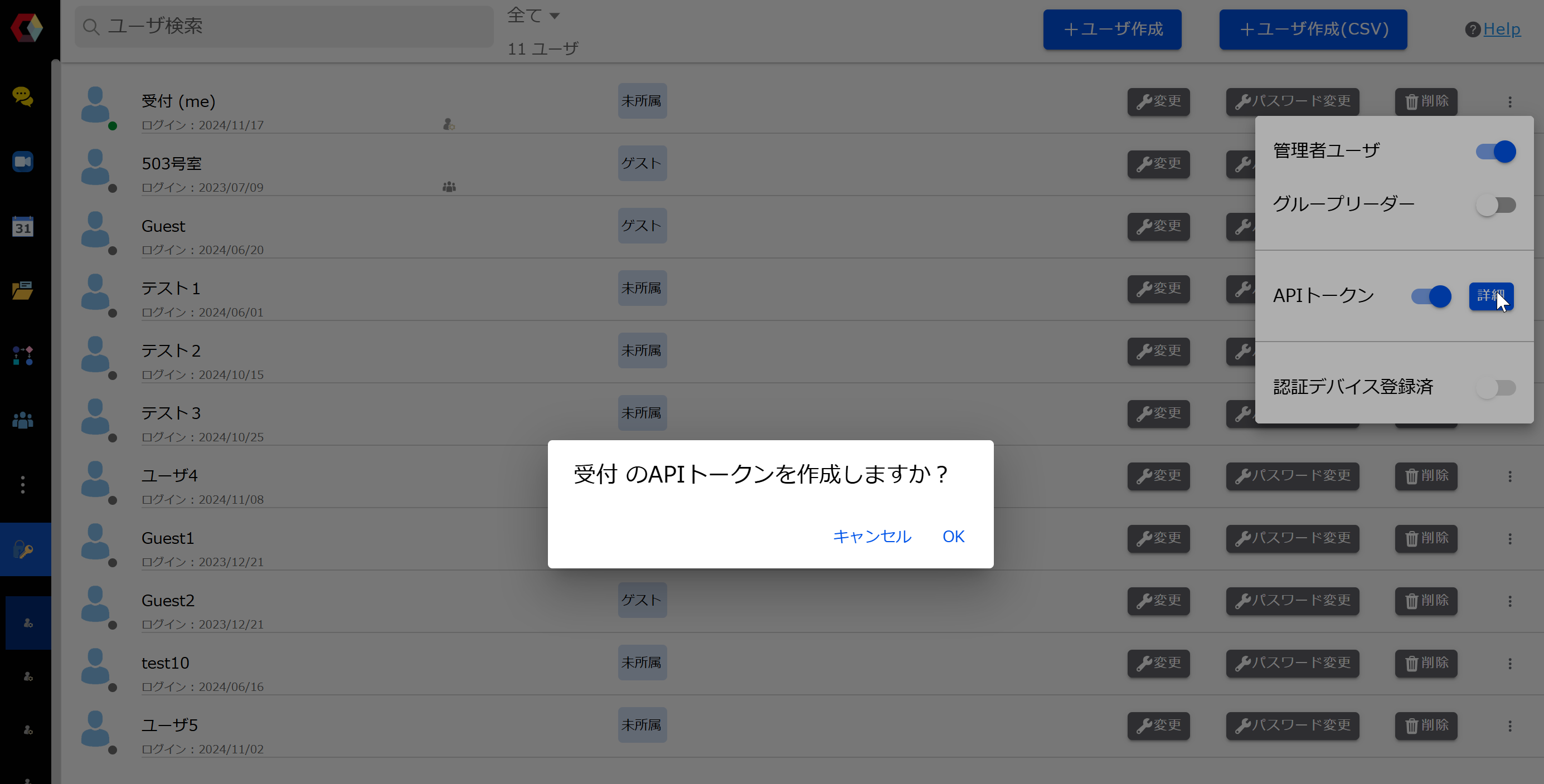
API Interface
JavaScript / curl command line is available as an API interface. Both of the following samples can retrieve meeting room registration information in JSON format.
In an environment where regular SSL is not applied, the server URL will be HTTP port 8080.
JavaScript
let config = {
"apiToken": "QIQVOSvRJHrQElDwj20x******",
"serverURL": "https://*****************"
}
let client = new CAMAPIClient(config);
let response = await client.getConferenceRooms();
curl command line
curl -H "x-cam-apiToken:token******" ${serverURL}/getConferenceRooms
Any HTTP access program
Any HTTP access program can be linked.
API Sample Screens
We have a sample URL where you can easily test API execution. If you would like to use it for testing, please contact us through Contact Us.
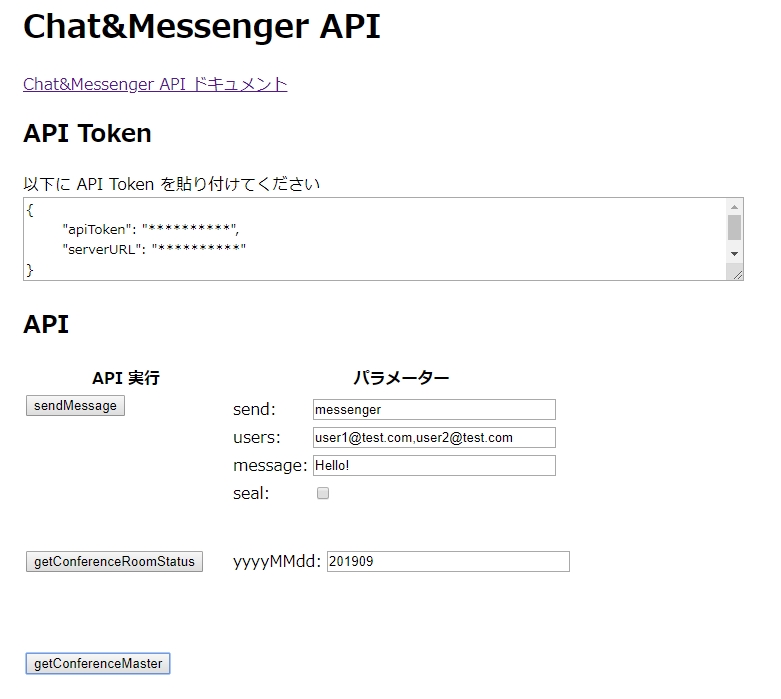
Message API
sendMessage
Send a message.
sample
Send by messenger with user ID
let response = await getAPIClient().sendMessage(
"messenger", // messenger を指定したダイレクトメッセージを指定
"Hello!", // 送信するメッセージ
false, // 封書は off
["user1@xxx.com", "user2@xxx.com"] // 宛先 Email アドレス
);
curl -H "x-cam-apiToken:token******" -d message="{\"panelName\":\"messenger\",\"message\":\"Hello\",\"isOpened\":false,\"generalPurposes\":{\"users\":\"user1@xxx.com,user2@xxx.com\"}}" ${serverURL}/sendMessage
Send by specifying a chat room
let response = await getAPIClient().sendMessage(
"ルーム名",
"Hello!",
false,
["user1@xxx.com", "user2@xxx.com"] // メンションとして Email アドレス指定
);
curl -H "x-cam-apiToken:token******" -d message="{\"panelName\":\"ルーム名\",\"message\":\"Hello\",\"isOpened\":false,\"generalPurposes\":{\"users\":\"user1@xxx.com,user2@xxx.com\"}}" ${serverURL}/sendMessage
argument (e.g. function, program, programme)
send |
|
users |
|
message |
|
seal |
|
sendMessages
Sends a batch message from a json file.
json files should be saved with the character code UTF8.
sample
curl -H "x-cam-apiToken:token******" -d @messages.json ${serverURL}/sendMessages
messages=[
{"message":"こんにちは 1","property":{"users":"user1@test.com,user2@test.com"}},
{"message":"こんにちは 2","property":{"users":"user1@test.com,user2@test.com"}},
{"message":"こんにちは 3","property":{"users":"user1@test.com,user2@test.com"}},
{"message":"こんにちは 4","property":{"users":"user1@test.com,user2@test.com"}},
]
messages=[
{"send": "test", "message":"テスト 1"}
]
Call API
createQuickCall
Quick Web Conference Create a URL.
sample
Create a quick meeting URL
let response = await getAPIClient().createQuickCall(
1624368868714, // クイック会議の期限(UnixTimeミリ)
"password!", // クイック会議のパスワードを指定
);
argument (e.g. function, program, programme)
expiredDate |
|
password |
|
User API
The User API only provides the curl command, and requires administrator privileges to run it.
exportUsers
User information will be downloaded in CSV format to the location specified by path.
sample
curl -H "x-cam-apiToken:token******" -d "path=exportUsers.csv" ${serverURL}/exportUsers
updateUsersCSV
Update user information in bulk from a CSV file. * See CSV format
sample
curl -H "x-cam-apiToken:token******" -F "file=@updateUsers.csv" ${serverURL}/updateUsersCSV
ChatRoom API
updateChatRoom
Create and update chatrums
sample
let chatRoom = {
"id":"1564831284702237059",
"name":"秘密会議"
"createUserId":"11u1pu9d32p8vuvjoZdd",
"adminUserId":"11u1pu9d32p8vuvjoZdd",
"isPublic":false,
"memberUids":{
"11u1pu9d32p8vuvjoZdd":true,
"1lmn7hoh3s1ja26fsazw":true,
"hhx10sfdv1jyou4la1ny":true,
"3400w9rfvs504c35dt99":true,
"309d32p8vuvjo5euuuwx":true
},
}
let response = await getAPIClient().updateChatRoom(chatRoom);
let errors = response['errors'];
if (errors) {
console.log(errors);
return;
}
console.log(response["chatRoom"]);
argument (e.g. function, program, programme)
chatRoom |
|
Schedule API
getConferenceMaster
Retrieves the master list of meeting rooms.
sample
let response = await getAPIClient().getConferenceMaster();
let errors = response['errors'];
if (errors) {
console.log(errors);
return;
}
console.log(response["conferenceRooms"]);
argument (e.g. function, program, programme)
without
getConferenceRoomStatus
Retrieve a list of meeting room reservations
sample
let response = await getAPIClient().getConferenceRoomStatus("201908");
let errors = response['errors'];
if (errors) {
console.log(errors);
return;
}
console.log(response["conferenceRoomStatus"]);
argument (e.g. function, program, programme)
yyyyMMdd |
|