Checking the type of a variable
Here is a sample program for checking variable types in Java.
Since Java is a statically typed language that declares types explicitly, there is no opportunity to check types to that extent. However, when using inherited objects, type checking may be necessary.
How to check types in Java instanceof Using operators, you can evaluate classes and interfaces with if statements, etc. as shown below.
variable instanceof class
variable instanceof interface
instanceof A class name or interface name can be specified on the right side of .instanceof The return value is a boolean; if true, the variable is the specified class or interface.
Chat&Messenger is groupware that integrates business chat, web conferencing, file sharing, schedule management, document management, conference room reservation, and attendance management in an easy-to-use manner. Perfect security for businesses,Available for free!
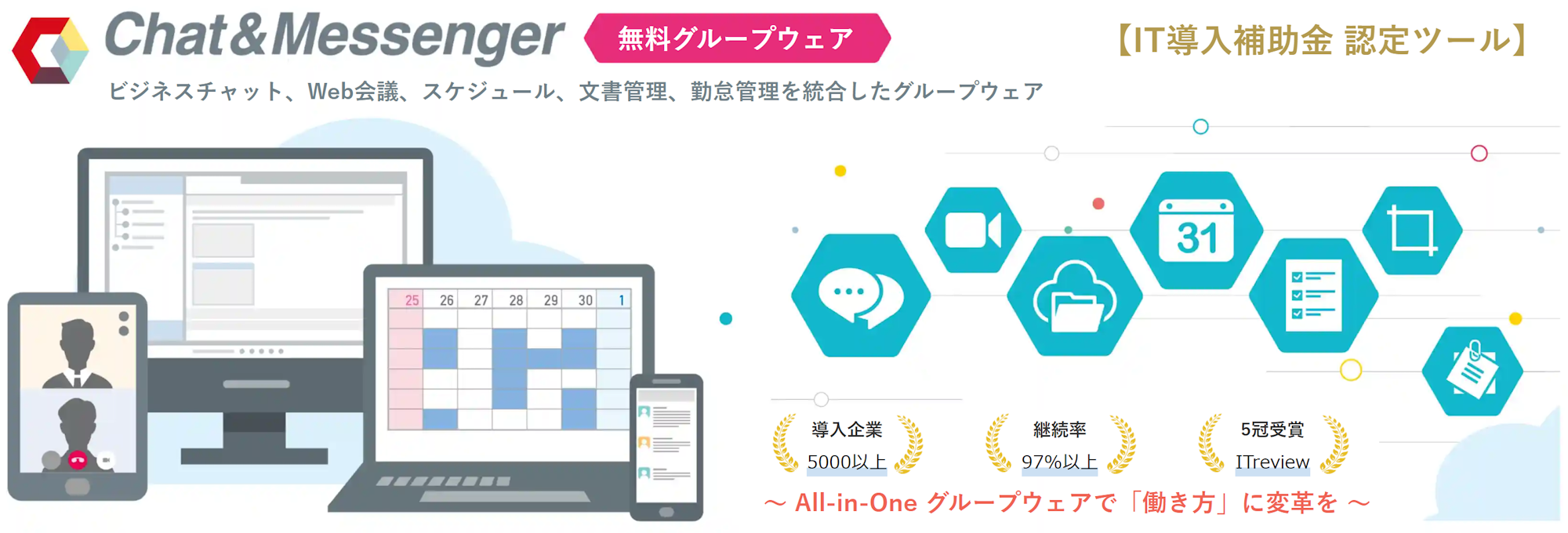
sample program
First, the simplest pattern. Returns true if variable i is Integer, false otherwise.
sample code
public static void integerSample() {
Integer i = 100;
boolean result = i instanceof Integer;
System.out.println(result);
}
output (result)
true
Since the variable i is an Integer, the result is true. However, this is too obvious to understand what instanceof is for, so we will make it a little more complicated.
Effective use of instanceof
One effective use of instanceof is to determine which child class the variable is.
sample code
In the sample code, we create an animal class Animal, a Dog class that inherits from the animal class, and a Cat class, and after creating an instance, set variables in the play method. instanceof The console output is branched based on the judgment.
class Animal {}
class Dog extends Animal {}
class Cat extends Animal {}
public class Main {
public static void main(String[] args) {
instanceofSample();
}
static void instanceofSample() {
Animal dog = new Dog();
play(dog);
Animal cat = new Cat();
play(cat);
}
static void play(Animal animal) {
if (animal instanceof Dog) {
System.out.println("お散歩する");
} else {
System.out.println("猫じゃらしで遊ぶ");
}
}
}
output (result)
お散歩する
猫じゃらしで遊ぶ
The key point is that in the play method, it is not known whether the animal passed as an argument is a dog or a cat.
instanceof Now, you can also check the subclass instances (Dog or Cat) contained within the superclass (Animal), so you can use it like this.
Check to see if a specific interface is implemented
You can check whether a specific interface is implemented for the variable targeted by instanceof. For example, you can use it like this.
sample code
In the sample code below, classes for Mr. Sato and Mr. Suzuki are created, and the interface Car is implemented assuming that only Mr. Suzuki owns a car. The goShopping() method assumes the process of going shopping, but it implements Car or branches the console output based on instanceof.
interface Car {}
class Sato {}
class Suzuki implements Car {}
public class Main {
public static void main(String[] args) throws Exception {
instanceofSample();
}
static void instanceofSample() {
Sato sato = new Sato();
goShopping(sato);
Suzuki suzuki = new Suzuki();
goShopping(suzuki);
}
static void goShopping(Object human) {
if (human instanceof Car) {
System.out.println("車で行く");
} else {
System.out.println("歩いて行く");
}
}
}
Output Results:
歩いて行く
車で行く
You can see that the process diverges between cases where Car is implemented and cases where it is not.